1. Overview
This portfolio aims to document the contributions that I made to the ExpenseTracker.
ExpenseTracker is a desktop expense tracking application developed to aid users in tracking their expenses and saving money. The user interacts with it using a Command Line Interface (CLI), and it has a Graphical User Interface (GUI) created with JavaFX library. It is written in Java, and has about 20k lines of code.
Some of its main features are:
-
Setting a budget for expenses.
-
Multiple user accounts.
-
Categorization of expenses.
-
Statistics for expenses.
2. Summary of contributions
This section highlights some of the contributions that I have made to Expense Tracker.
-
Major enhancement: added the ability to have a budget on expenses.
-
Function: It informs the user if the their spendings exceed a set budget
-
Justification: This is a core feature as it allows the user to know when they are spending too much money, the main purpose of an expense tracker.
-
Highlights: This enhancement affects existing commands and commands to be added in future. It required an in-depth analysis of software design alternatives. The implementation was challenging as it required changes to existing commands and the existing implementation of the main
ExpenseTracker
class.
-
-
Minor enhancements:
-
Added a
setRecurrenceFrequency
command that allows the user to reset their available spending at a frequency of their choice. -
Added a
setCategoryBudget
command that allows the user to set budgets based on their category of expenses.
-
-
Code contributed: [Code]
-
Other contributions:
-
Project management:
-
Created the organisation repository, set up issue tracker and milestones.
-
-
Documentation:
-
Community:
-
Tools:
-
Created a script to pull code from main fork to local fork. #17
-
-
3. Contributions to the User Guide
Given below are sections I contributed to the User Guide. They showcase my ability to write documentation targeting end-users. |
Setting a total budget: setBudget
Sets a total budget. Available spending is defined as the total amount of expenses you can add before you exceed your budget. The Expense Tracker will warn you when adding an expense results in your available spending exceeding the total budget that is set to prevent yourself from overspending. The warning is shown below.
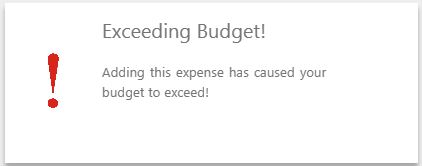
Format: setBudget MONEY_WITH_TWO_DECIMAL_PLACES
|
Setting a recurrence frequency for total budget: setRecurrenceFrequency
Sets the recurrence frequency for resetting the available spending of the total budget of Expense Tracker.
If you draw a salary/have an allowance, this would allow you to track the portion of money you have spent against your salary/allowance of the week/month. This ensures that you do not overspend on a certain day, causing you to not have enough money until you draw your next salary/allowance.
A diagram of a use case and its detailed explanation is given below:
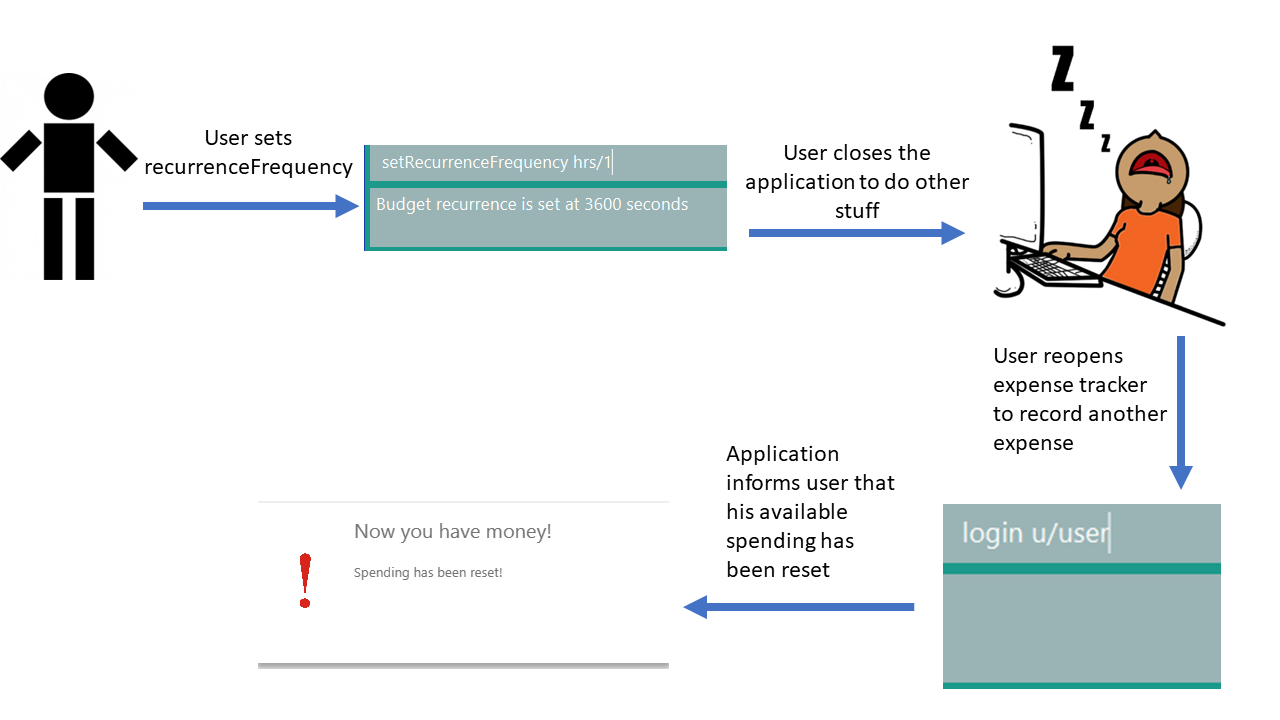
-
You type in the command
setRecurrenceFrequency hrs/24
as you want your available spending to reset every day when you receive your allowance. -
You close the application.
-
You reopen the application on the next day to record another expense.
-
Your available spending will now be reset as it is past the set frequency of one day. A notification will be shown to inform you that this is done.
Format: setRecurrenceFrequency [hrs/HOURS] [min/MINUTES] [sec/SECONDS]
|
Setting a Category Budget: setCategoryBudget
Sets a Budget to limit expenses on a certain category. If adding an expense causes the available spending of that category to exceeds Category budget, a warning will be displayed.
For more details and caveats for this feature, please refer to the user guide.
4. Contributions to the Developer Guide
Given below are sections I contributed to the Developer Guide. They showcase my ability to write technical documentation and the technical depth of my contributions to the project. |
Budgeting
This group of features allows the user to set budgets for their expenses.
Available spending is defined as the total amount of expenses you can add before you exceed your budget. If the user’s spending exceeds their available spending for the budget, a warning will be shown to the user.
The current implementation for budgeting and its related features are described in the sections below.
Setting a Budget
This feature allows the user to set a budget for Expense tracker.
Given below is a sequence diagram and step by step explanation of how Expense Tracker executes when a user sets a budget
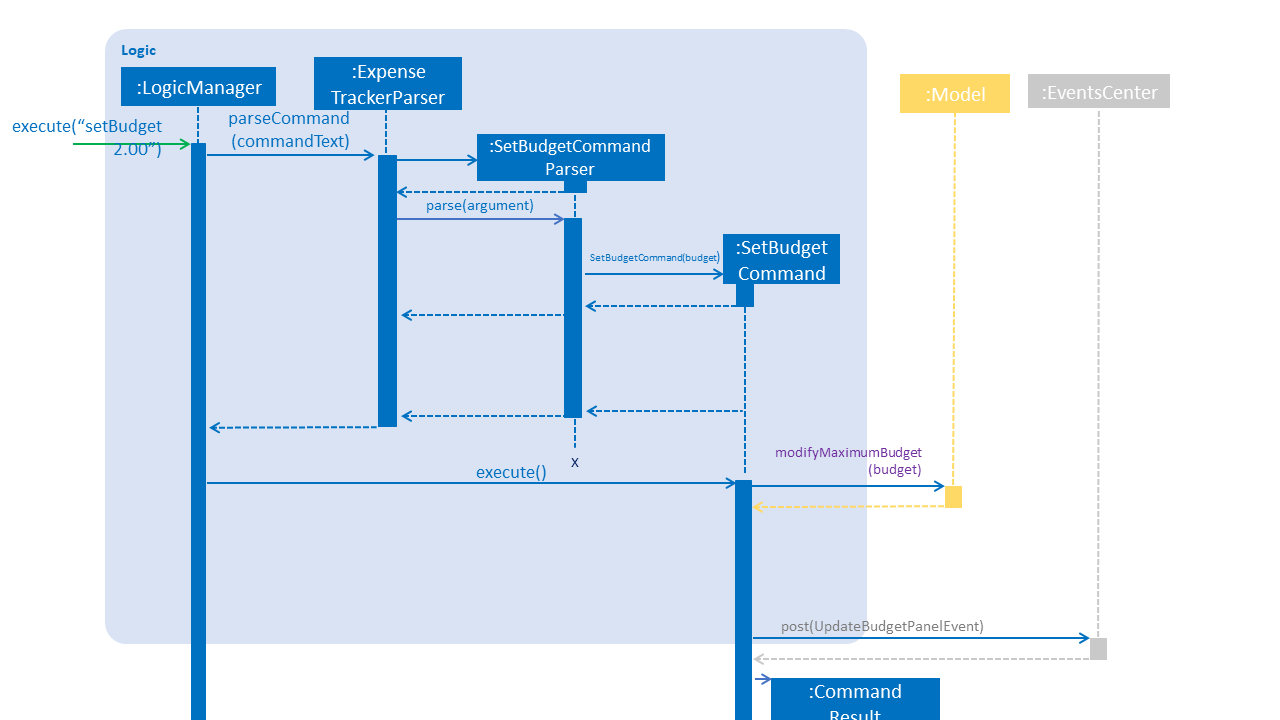
-
User enters command
setBudget 2.00
. -
The command is received by
ExpenseTrackerParser
, which then creates aSetBudgetCommandParser
Object and callsSetBudgetCommandParser#parse()
method. -
SetBudgetCommandParser#parse()
will then return abudget
ofdouble
type. It will then create aSetBudgetCommand
Object withbudget
as a parameter would be created and returned toLogicManager
. -
LogicManager
then callsSetBudgetCommand#execute()
, which callsModelManager#modifyMaximumBudget
to update the maximum budget of Expense Tracker. -
LogicManager
will then callEventsCenter#post()
to update the UI, displaying the updated budget.
Setting a recurring Budget
This feature allows the user’s available spending to reset every user defined frequency.
The implementation for this feature is describe in the two sections below.
1. Setting the recurrence frequency
This section explains the implementations of setRecurrenceFrequency
command.
-
Recurrence time is set by
setRecurrenceFrequency
. If it has not been set before, the next recurrence time will be set tocurrentTime
+recurrenceFrequency
. This is shown in the code snippet below.
public void setRecurrenceFrequency(long seconds) {
this.numberOfSecondsToRecurAgain = seconds;
this.nextRecurrence = LocalDateTime.now().plusSeconds(seconds);
}
-
If it has already been set, the timing will be updated on the next occurrence time. This is shown in the code snippet below.
if (LocalDateTime.now().isAfter(this.nextRecurrence)) {
this.previousRecurrence = LocalDateTime.now();
this.nextRecurrence = LocalDateTime.now().plusSeconds(this.numberOfSecondsToRecurAgain);
// rest of the implementation
{
For more details on explanation on how Expense Tracker executes when a user sets a recurrence frequency and its sequence diagram, please refer to the Developer Guide.
2. Resetting available spending.
This section explains the implementations of the recurring budget.
Every time the user logs in, Expense Tracker will check if the available spending should be reset. Sequence diagram of available spending resetting is given below.
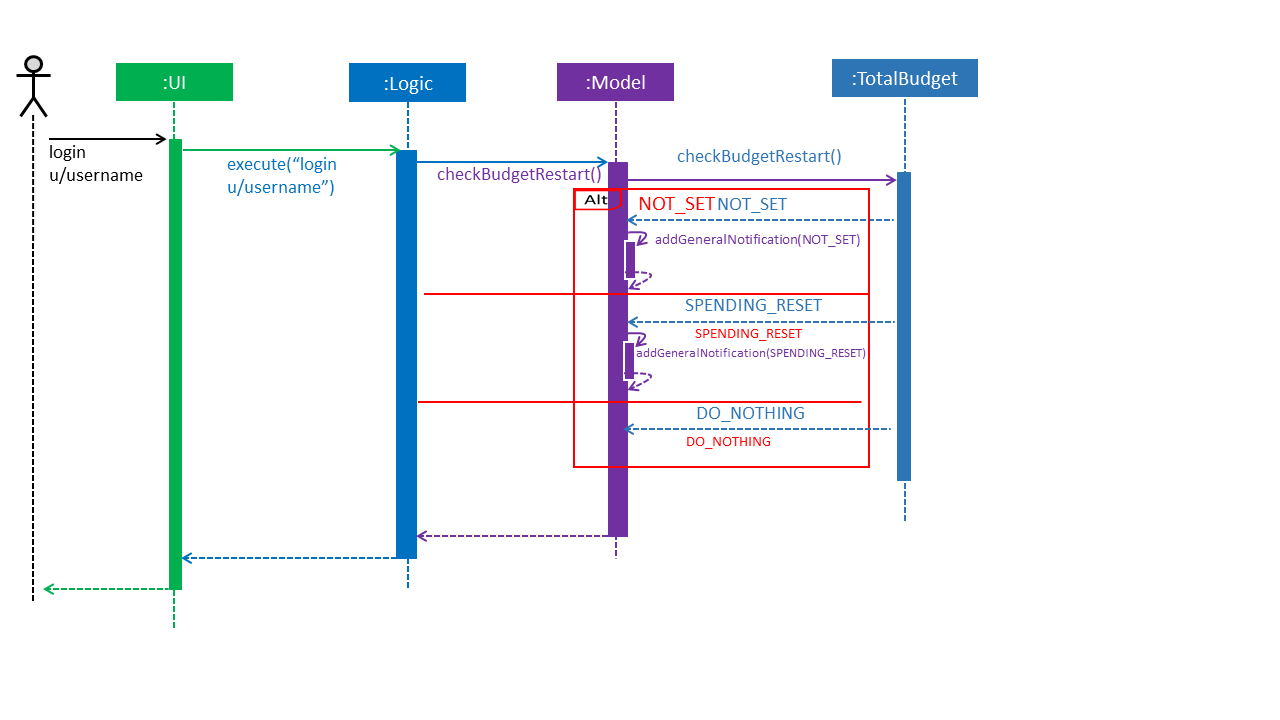
Execution steps of resetting available spending are as follows:
-
User logs in, which causes the
login
command to execute inLogic
.Logic
callsLogic#execute()
to execute the command on theModel
. -
login
command executes in theLogic
, which callsLogic#execute()
to execute the command onModel
-
Model
callsModel#checkBudgetRestart()
, which in turn callsTotalBudget#checkBudgetRetart()
to check if the available spending is to be reset. -
TotalBudget#checkBudgetRestart()
either returnNOT_SET
orSPENDING_RESET
, which will add their respective notifications. It will also returnDO_NOTHING
, which will results inModel
to continue its execution.
Setting a Budget by Category
An extension to the budget feature, this allows the user to divide their budget based on categories. Users can allocate parts of their budget to certain categories. Refer to the Developer Guide for more details.
Setting budgets for different time frames (Proposed)
Users can now set budgets for different time frames. For example, a user can have a monthly and weekly budget. This is to allow a user to segment his spending by weeks. Thus, even if the user has spent over the budget for this week, he could potentially spend lesser in the next week to make up for his overspending and keep within his budget for the month. This is illustrated by the images below.
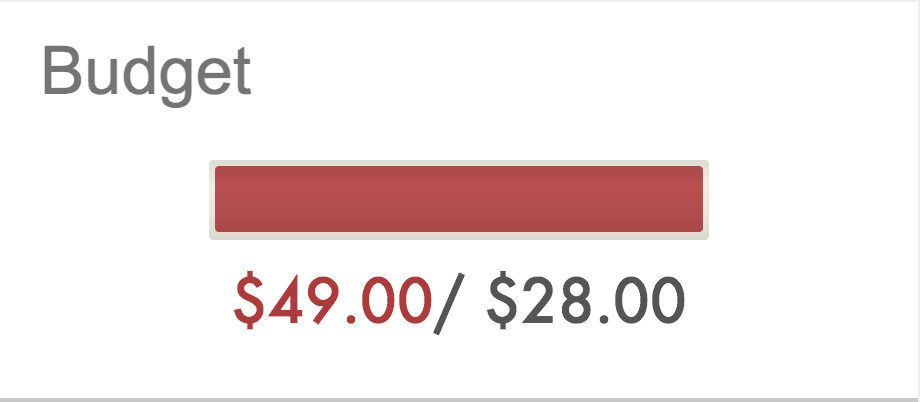
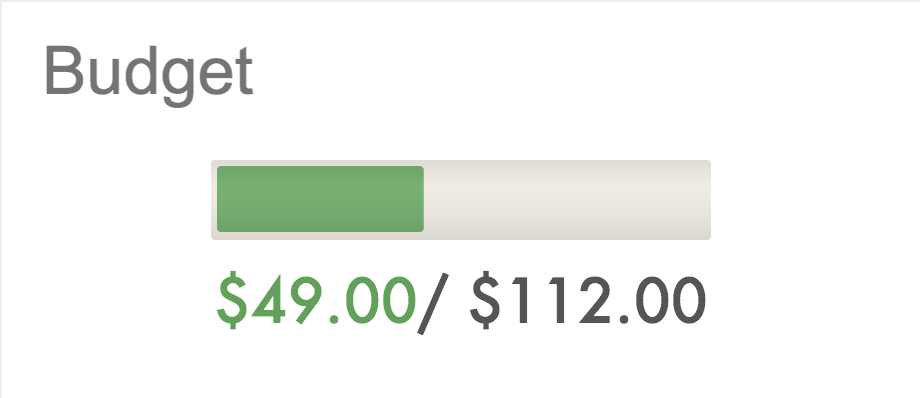
The first image shows that the user has spent over his budget for the week, while the second image shows that the user still has available spending for the month.
Design Considerations
This section provides alternative design patterns that we have considered for features relating to budgeting.
Aspect: How recurrence is checked
-
Alternative 1 (current choice): Calling of method when the user logs in
-
Pros: Closely coupled with logging in.
-
Cons: Encapsulation sacrificed due to close coupling with other classes and methods.
-
-
Alternative 2: Dispatching an event every time the user logs in
-
Pros: Easy to implement
-
Cons: Possible for other implementations to cause a recurrence check. As the recurrence check is closely tied to logging in, this should not be possible
-